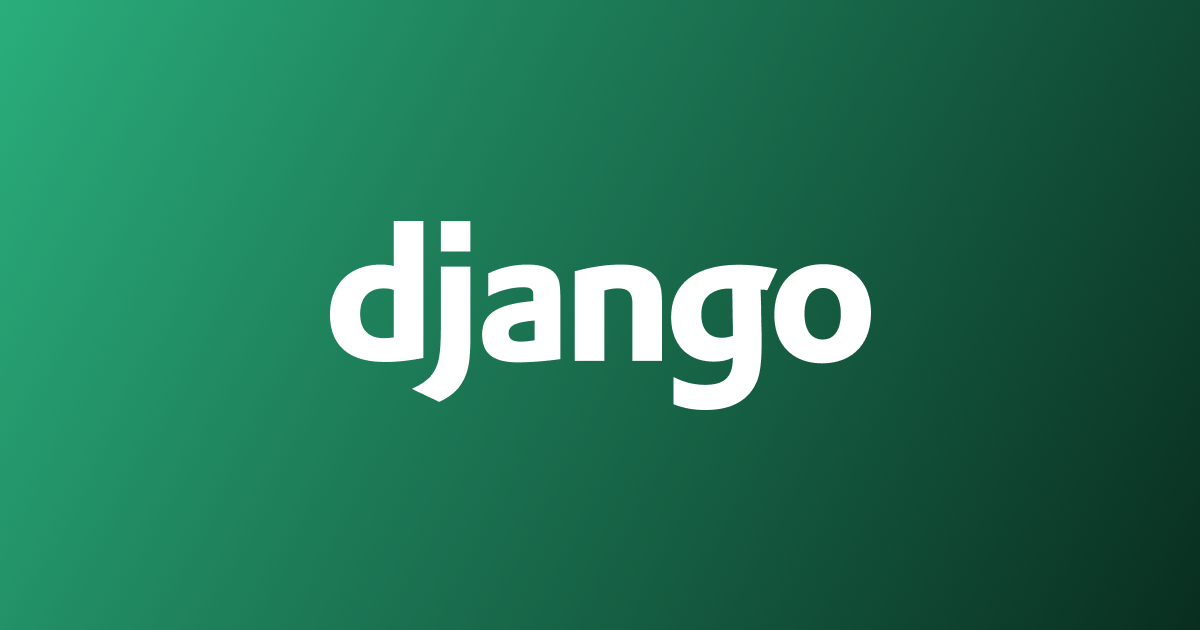
Django: Revolutionizing Web Development with Python
Django is a powerful and popular Python web framework that simplifies the process of developing web applications. It follows the model-view-controller (MVC) architectural pattern and encourages the use of reusable components, making it an excellent choice for building scalable and maintainable web applications. Django's robustness, scalability, and extensive built-in features have made it a preferred framework for developers worldwide.
In this article, we will explore the key features of Django, its benefits, and how to get started with Django development. We will also provide code examples to illustrate various aspects of Django's functionality. Whether you're a beginner or an experienced developer, this article will serve as a comprehensive guide to Django web development.
Getting Started with Django
To begin working with Django, you'll need to have Python installed on your machine. Django is compatible with Python 3.6 or later versions, so make sure you have the appropriate Python version installed. You can download Python from the official website and follow the installation instructions for your operating system.
Once Python is installed, you can proceed with installing Django. Open a terminal or command prompt and use the following command:
pip install django
This command will install Django and its dependencies on your system. After the installation is complete, you can verify the installation by running the following command:
django-admin --version
If Django is installed correctly, you will see the version number displayed in the terminal.
Creating a Django Project
Now that Django is installed, let's create a new Django project. Django provides a command-line utility called django-admin
that helps manage various aspects of Django projects. To create a new project, run the following command:
django-admin startproject myproject
This command creates a new directory named myproject
, which will serve as the project's root directory. Inside the myproject
directory, you will find a manage.py file and another directory with the same name as your project. The manage.py file is used to interact with your Django project, and the inner directory contains the project's settings and configuration files.
Running the Development Server
To test your newly created Django project, navigate to the project's root directory (myproject
) using the terminal or command prompt. Once inside the directory, run the following command:
python manage.py runserver
This command starts the development server, which is a lightweight web server included with Django. By default, the server runs on http://127.0.0.1:8000/
. Open your web browser and enter this address to see the default Django welcome page.
Congratulations! You have successfully set up a Django project and started the development server. Now let's dive deeper into Django's features and explore how it can make your web development journey easier.
Key Features of Django
Django offers a wide range of features that simplify the web development process. Let's take a look at some of the key features that make Django stand out from other frameworks:
1. Object-Relational Mapping (ORM)
Django provides a powerful and intuitive Object-Relational Mapping (ORM) layer that allows you to interact with your database using Python objects. The ORM eliminates the need to write complex SQL queries and handles the database operations for you. It supports multiple database backends, including PostgreSQL, MySQL, SQLite, and Oracle.
Here's an example that demonstrates how to define a simple model in Django's ORM:
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=100)
author = models.CharField(max_length=100)
publication_date = models.DateField()
In this example, we define a Book
model with three fields: title
, author
, and publication_date
. Django's ORM will automatically create the corresponding database table and handle CRUD operations for this model.
2. Admin Interface
Django provides an automatic admin interface that allows you to manage your application's data without writing any additional code. The admin interface is fully customizable and provides an easy-to-use interface for creating, updating, and deleting records. It also includes features such as search, filtering, and sorting.
To enable the admin interface for your models, you need to register them in the admin.py file:
from django.contrib import admin
from .models import Book
admin.site.register(Book)
Once you've registered your models, you can access the admin interface by running the development server and visiting http://127.0.0.1:8000/admin/
. You will be prompted to log in with the credentials of a superuser that you can create using the createsuperuser
command.
3. URL Routing and View Handling
Django follows the URL routing mechanism, which allows you to map URLs to corresponding view functions. Views handle the logic for processing requests and returning responses. Django provides a simple and elegant way to define URL patterns and associate them with views.
Here's an example that demonstrates how to define a URL pattern and associate it with a view:
from django.urls import path
from .views import home_page
urlpatterns = [
path('', home_page, name='home'),
]
In this example, we define a URL pattern that matches the root URL (http://127.0.0.1:8000/
) and associates it with the home_page
view function. The name
parameter is used to uniquely identify the URL pattern, which can be useful for generating URLs dynamically.
4. Template Engine
Django comes with a powerful template engine that allows you to separate the presentation logic from the business logic in your web application. Templates are written using HTML, with the ability to embed Python code and access data passed from views.
Here's a simple example that demonstrates the use of Django templates:
<!-- templates/home.html -->
<html>
<head>
<title>Home Page</title>
</head>
<body>
<h1>Welcome to my Django website!</h1>
{% if user.is_authenticated %}
<p>Hello, {{ user.username }}!</p>
{% else %}
<p>Please log in to continue.</p>
{% endif %}
</body>
</html>
In this example, we have a basic HTML template that displays a welcome message. The {% if %}
statement checks if the user is authenticated and displays a personalized message accordingly. Django's template engine will render this template with the appropriate data.
5. Form Handling
Django provides a powerful form handling mechanism that simplifies the process of working with HTML forms. Django forms handle form validation, data binding, and error handling, making it easy to build complex forms with minimal effort.
Here's an example that demonstrates how to define a simple form in Django:
from django import forms
class ContactForm(forms.Form):
name = forms.CharField(max_length=100)
email = forms.EmailField()
message = forms.CharField(widget=forms.Textarea)
In this example, we define a ContactForm
class with three fields: name
, email
, and message
. Django's form handling automatically generates the necessary HTML form elements and performs validation when the form is submitted.
Django Terminal Commands
Django provides a command-line utility called manage.py
that allows you to perform various tasks related to your Django project. Here are some commonly used terminal commands and their explanations:
1. Starting a New Django Project
To create a new Django project, use the following command:
python manage.py startproject project_name
This command creates a new Django project with the given project_name
. The project structure will be set up with necessary files and directories.
Example:
python manage.py startproject myproject
2. Running the Development Server
To start the development server and run your Django project locally, use the following command:
python manage.py runserver
This command starts the development server, and by default, the server runs on http://127.0.0.1:8000/
. You can access your Django application by opening this URL in your web browser.
Example:
python manage.py runserver
3. Creating a New Django App
Django projects consist of multiple apps. To create a new app within your Django project, use the following command:
python manage.py startapp app_name
This command creates a new Django app with the given app_name
. The app will have its own directory structure and files.
Example:
python manage.py startapp myapp
4. Applying Database Migrations
Django uses database migrations to manage changes to your database schema. To apply pending migrations, use the following command:
python manage.py migrate
This command applies any pending database migrations and synchronizes the database schema with the current state of your Django models.
Example:
python manage.py migrate
5. Creating Database Migrations
When you make changes to your models, you need to create database migrations to reflect those changes in the database. Use the following command to create a new migration:
python manage.py makemigrations
This command analyzes your models and creates new migration files that capture the changes to be applied to the database.
Example:
python manage.py makemigrations
6. Interacting with the Django Shell
Django provides an interactive shell that allows you to interact with your Django project and perform operations programmatically. Use the following command to start the Django shell:
python manage.py shell
This command opens the Django shell, where you can import Django modules, access your models, and execute Python code.
Example:
python manage.py shell
These are just a few examples of the Django terminal commands you can use to manage your Django projects. Django's manage.py
utility provides a wide range of commands for tasks like creating superusers, running tests, and more. You can explore the Django documentation for a complete list of available commands.
Remember to always run these commands in the root directory of your Django project, where the manage.py
file is located.
Conclusion
Django is a powerful and feature-rich Python web framework that simplifies the process of developing web applications. In this article, we explored the key features of Django, including its Object-Relational Mapping (ORM), admin interface, URL routing, template engine, and form handling. We also provided code examples to illustrate how to get started with Django development.
With Django's extensive documentation, vibrant community, and vast ecosystem of reusable packages, you'll find ample resources and support to build robust and scalable web applications. Whether you're a beginner or an experienced developer, Django's intuitive design and comprehensive features make it an excellent choice for web development.
Start your Django journey today and unlock the potential to build dynamic and engaging web applications with ease!